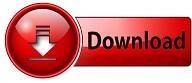
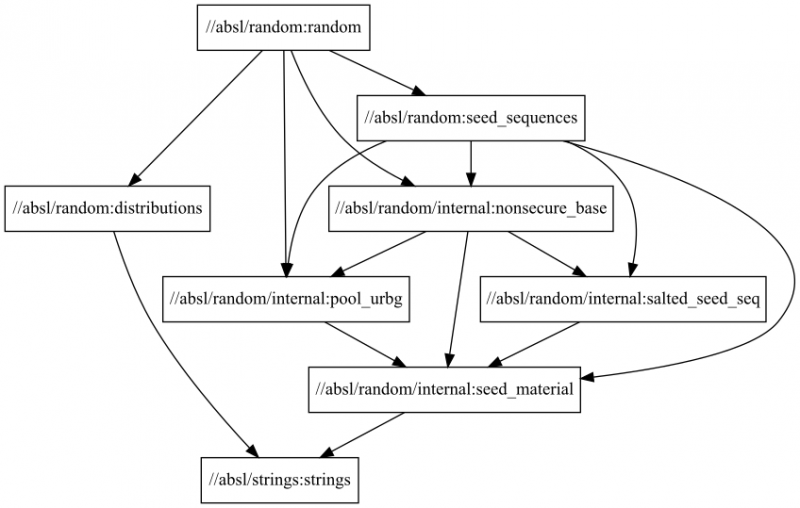
The get_min_value function is used to find the minimum value node in the right subtree of a node with two children, which is used during deletion. It handles different cases, such as deleting a node with no children (leaf node), deleting a node with one child (either left or right), or deleting a node with two children. The deletion operation is also recursive. Here root represents the root of the binary tree, and key represents the value of the node to be deleted. An example implementation of an insertion operation for a binary tree: def insert(root, key): if root is None: return TreeNode(key) else: if key root.key: root.right = delete(root.right, key) else: if root.left is None: temp = root.right root = None return temp elif root.right is None: temp = root.left root = None return temp temp = get_min_value(root.right) root.key = temp.key root.right = delete(root.right, temp.key) return root def get_min_value(root): while root.left is not None: root = root.left return root The insertion operation typically involves finding the correct position in the tree to place the new node based on its value. Insertion is the process of adding a new node to the binary tree. right: Represents the right child node of the current node.
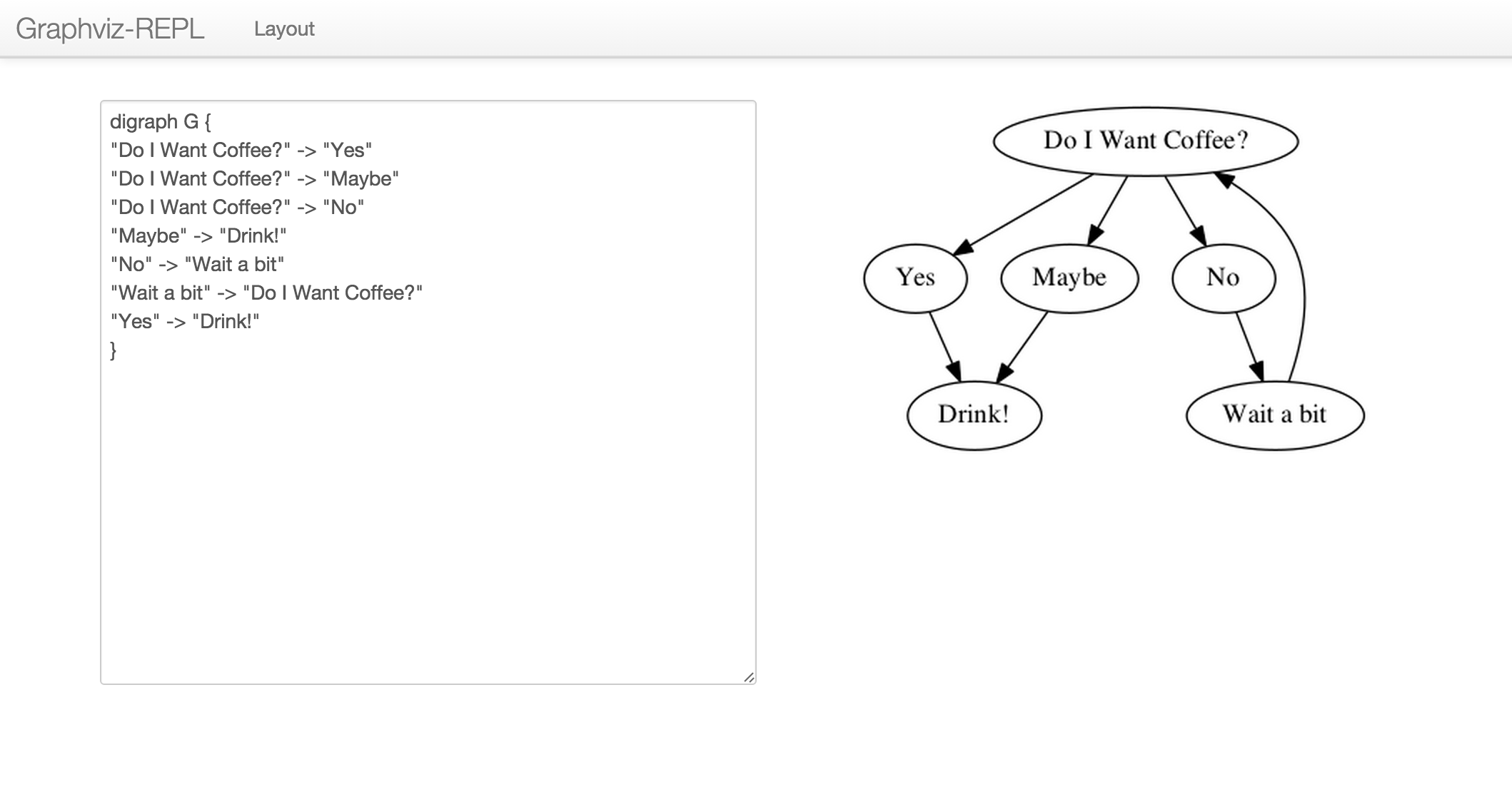
left: Represents the left child node of the current node.key: Represents the value or key stored in the node.In the above implementation, the TreeNode class has three attributes: Here is an example implementation of a binary tree node: class TreeNode: def _init_(self, key): self.key = key self.left = None self.right = None In Python, binary trees can be implemented using classes, with each node in the tree represented as an object of the class. This article will explore how binary trees are implemented in Python and discuss common operations such as insertion, deletion, and traversal. In Python, binary trees can be implemented using classes and objects, providing a flexible and efficient way to store, manipulate, and search for data in a tree-like structure. Binary trees are fundamental data structures in computer science that are used to represent hierarchical relationships between objects.
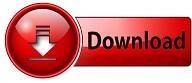